

We can use add method, specifying an index where to add the element followed by the element. Saturn Adding element to middle of a LinkedList Planets.addAll(listOf("Jupiter", "Saturn")) In the following example, we are adding more planets to our list of planets using addAll method.

We can use method addAll to add a list of elements to linked list. Pluto Adding elements to an existing LinkedList In the following example, we are iterating through linked list of planets and printing each planet. We can use for loop to iterate through a LinkedList in Kotlin. In the following example, we are removing the last planet "Pluto" from the planet list using removeLast function. We can remove element from the end of the LinkedList using removeLast function.
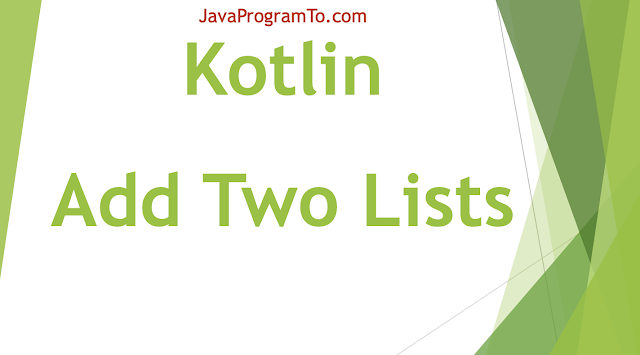
Planets.addAll(listOf("Mercury", "Venus", "Earth", "Pluto")) In the following example, we are removing the first planet "Mercury" from the planet list using removeFirst function. We can remove element from the start of the LinkedList using removeFirst function. In the following example, we used addLast method to add Pluto planet to the end of the LinkedList. We can add element to the end of the linked list, using addLast method. In the following example we used addFirst method to add Mercury planet to the top of the LinkedList. We can add element to the start of the linked list, using addFirst method. In the following example, we are retrieving the last element and displaying it. We can get the last element of the LinkedList using last member variable. Println("First planet = " + planets.first) In the following example, we are retrieving the first element and displaying it. We can get the first element of the LinkedList using first member variable. Planets.addAll(listOf("Earth", "Venus", "Mars")) In the following example, we created a linked list of planets. We can create a linkedlist in Kotlin using. It is handled by the the underlying collections framework.
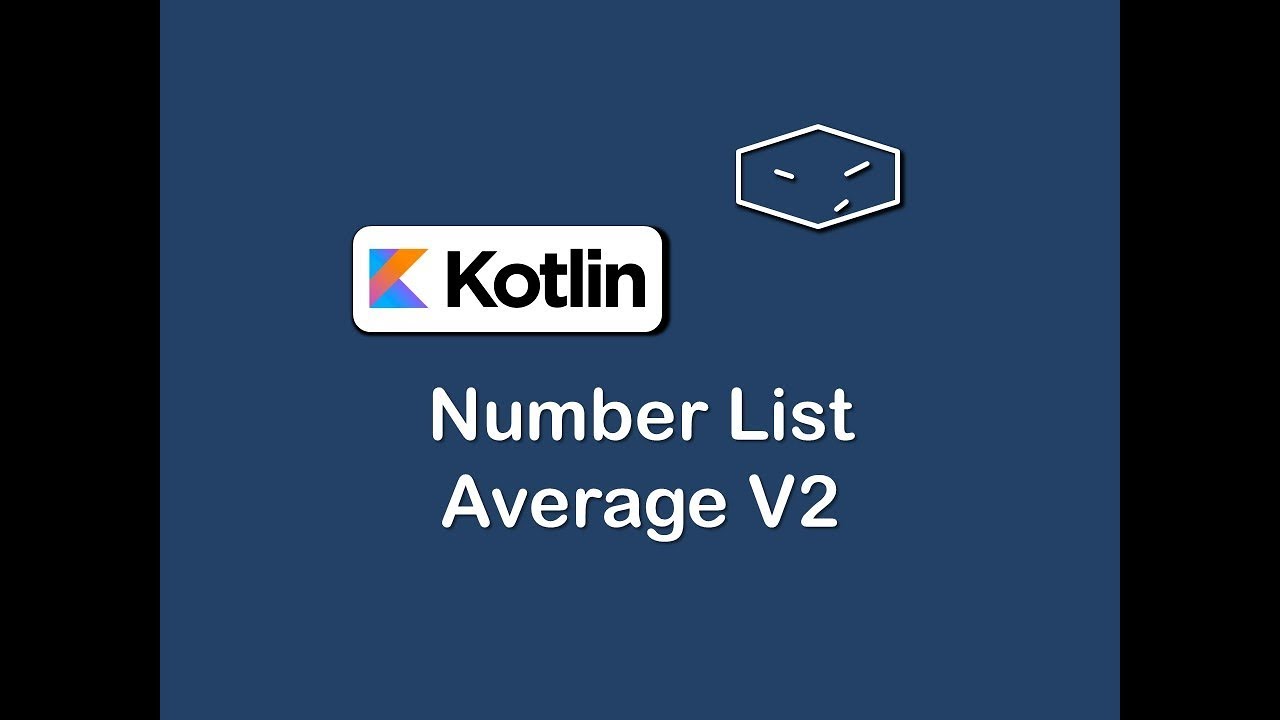
So we can add elements and the size will dynamically increase, and if we remove elements, the size will dynamically reduce. Benefit of linked list is that it is faster to insert and delete an element from LinkedList, it doesn't need rearrangement of entire underlying array. It belongs to the Java collections framework. It implements both the List and Deque interface. LinkedList provides a doubly linked list data structure implementation.
